golang strings can be seen as a collection of characters. A string can be of length 1 (one character), but its usually longer. A string is always written in double qoutes.
This means a string variable in golang can hold text: words, sentences, books
Programming languages have variables. These variables have a data type A variable can be of the data type string.
String example
String variable
In the example below we use golang to print text. First define a
string variable, then output the variable with the function
Printf()
. Make sure you have the package "fmt"
imported.
package main
import "fmt"
func main() {
var str1 = "This is a string variable!"
.Printf(str1)
fmt}
Multiple lines
In Go programming, there are two ways to print multiple lines.
- Method 1. call display function
Println
x times, - Method 2. use the newline character
\n
inside the string
Both of these are common in programming.
package main
import "fmt"
func main() {
var str1 = "This is a string variable!"
// 1: function calls
.Println(str1)
fmt.Println(str1)
fmt
// 2: use newline character
.Printf("Hello World\nI am Go")
fmt}
This is a string variable!
This is a string variable!
Hello World
I am Go
Program exited.
Note: The zero value of type string is a string of zero length, that is an empty string
""
.
String index
The contents of the string (bytes) can be obtained by standard
indexing, with an index written in parentheses []
, and the
index count from 0.
[0]
str[i-1]
str[len(str)-1] str
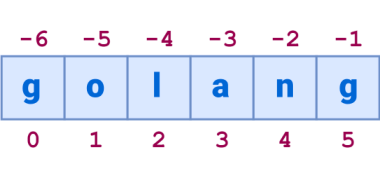
It is important to note that this conversion scheme is valid only for strings of pure ASCII codes (not Japanese, Chinese etc).
package main
import (
"fmt"
)
func main() {
:= "Go string example"
s for k, v := range s {
.Printf("k:%d,v:%c == %d\n", k, v, v)
fmt}
}
k:0,v:G == 71
k:1,v:o == 111
k:2,v: == 32
k:3,v:s == 115
k:4,v:t == 116
k:5,v:r == 114
k:6,v:i == 105
k:7,v:n == 110
k:8,v:g == 103
k:9,v: == 32
k:10,v:e == 101
k:11,v:x == 120
k:12,v:a == 97
k:13,v:m == 109
k:14,v:p == 112
k:15,v:l == 108
k:16,v:e == 101
Note Getting the address of a byte in the string is illegal, for example: &str [i].
The string of multiple lines in the code can be spliced in the following ways.
Direct-use operators
:= "Beginning of the string " + "second part of the string" str
The operator +=
can also be used for strings:
:= "hel" + "lo, "
s += "world!"
s .Println(s) fmt
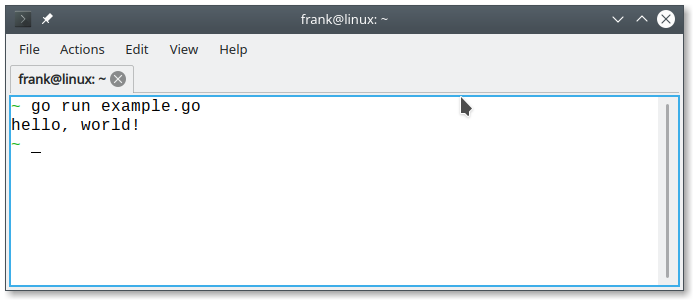
The general comparison operators (==
,!=
,
<
, <=
,>=
,
>
) implement the comparison of strings by byte
comparison in memory. You can get the byte length of the string by
function len()
, for example: len(str)
.
String join
To join strings, you can use the package strings
which
has the method .Join()
.
package main
import (
"fmt"
"strings"
)
func main() {
:= strings.Join([]string{"hello", "world"}, ", ")
s .Println(s)
fmt}
hello, world
Program exited.
Print variables
You can pass variables of different data types into the string. The example below passes an integer and a string data type:
package main
import (
"fmt"
)
func main() {
.Printf("%d:%s", 2016, "year")
fmt}
2016:year
Program exited.
Video
Video tutorial below
Exercises
- Create a program with multiple string variables
- Create a program that holds your name in a string.