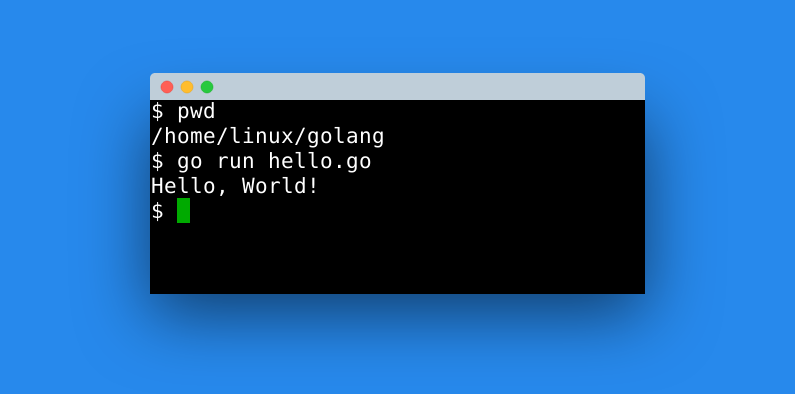
Golang Tutorial: Your First Program
Welcome to the Golang Tutorial! In this tutorial, we will be creating your very first program in Go.
Quick Start
Create a “Hello, World!” App
One of the easiest programs to create is a program that simply displays “Hello, World!” to the console. Here’s how you can create this program:
- Create a new file called
hello.go
. - Copy the following code into the file:
package main
import "fmt"
func main() {
.Println("Hello, World!")
fmt}
The code above does the following: - The package main
line tells Go that this is a main package and that it can be compiled
into an executable program. - The import "fmt"
line imports
the fmt
package, which provides functions for formatting
and printing strings. - The func main() { ... }
block is
where the program starts. This is where you can write your code.
- Save the file.
Run Your Program
Now that you’ve created your “Hello, World!” program, you can run it with the following steps:
- Open a terminal or command prompt.
- Navigate to the directory where your
hello.go
file is located. - Run the following command:
go run hello.go
.
You should see the message “Hello, World!” printed to the console.
Video Tutorial
Prefer watching a video? Check out this tutorial on YouTube:
Exercises
Now that you’ve created your first program, it’s time to practice with some exercises. Here are two exercises you can try:
- Create a program that displays your name.
- Create a program that displays your address.
Once you’re done, you can download the answers from this link.
Happy coding!