golang building a simple server is pretty simple, here are three ways to rewrite it from the ground up internally
- The first program uses the default DefaultServeMux and default Server structure.
- The second will use a custom handler
- The third will use a custom Server structure
In terms of execution efficiency, the third one is the fastest to execute, after all, it’s unencapsulated.
golang http server
The program below starts a web server at your localhost port 8080. It has one route, namely the / route. If you open the url in the browser, it will output “version 1”.
package main
import (
"io"
"log"
"net/http"
)
func main() {
// Set routing rules
.HandleFunc("/", Tmp)
http
//Use the default DefaultServeMux.
:= http.ListenAndServe(":8080", nil)
err if err != nil {
.Fatal(err)
log}
}
func Tmp(w http.ResponseWriter, r *http.Request) {
.WriteString(w, "version 1")
io}
This is the most common way to create a server without thinking too much about it!
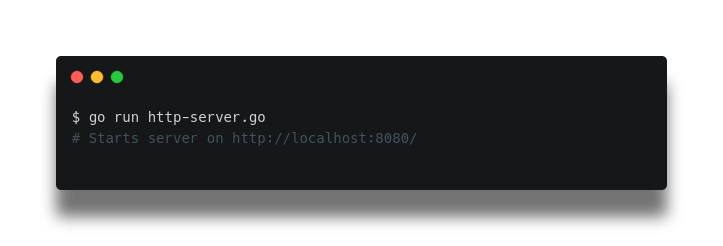
Routes
You can create more than one route. Mux is used to control access to the route by implementing a Handler registration into Mux.
package main
import (
"io"
"log"
"net/http"
)
type myHandler struct{}
func (*myHandler) ServeHTTP(w http.ResponseWriter, r *http.Request) {
.WriteString(w, "URL: "+r.URL.String())
io}
func Tmp(w http.ResponseWriter, r *http.Request) {
.WriteString(w, "version 2")
io}
func main(){
:= http.NewServeMux()
mux
// Register routes and register handlers in this form.
.Handle("/",&myHandler{})
mux
.HandleFunc("/tmp", Tmp)
mux
//http.ListenAndServe uses the default server structure.
:= http.ListenAndServe(":8080", mux)
err if err != nil {
.Fatal(err)
log}
}
golang http router
Listening and implementing http services with a custom server. In the
examle below we define a route like mux["/tmp"]
.
package main
import (
"io"
"log"
"net/http"
"time"
)
//Define a map to implement routing table.
var mux map[string]func(http.ResponseWriter , *http.Request)
func main(){
:= http.Server{
server : ":8080",
Addr: &myHandler{},
Handler: 5*time.Second,
ReadTimeout}
= make(map[string]func(http.ResponseWriter, *http.Request))
mux ["/tmp"] = Tmp
mux:= server.ListenAndServe()
err if err != nil {
.Fatal(err)
log}
}
type myHandler struct{}
func (*myHandler) ServeHTTP(w http.ResponseWriter, r *http.Request){
// Implement route forwarding
if h, ok := mux[r.URL.String()];ok{
//Implement route forwarding with this handler, the corresponding route calls the corresponding func.
(w, r)
hreturn
}
.WriteString(w, "URL: "+r.URL.String())
io}
func Tmp(w http.ResponseWriter, r *http.Request) {
.WriteString(w, "version 3")
io}
The above three ways are actually the unpacking of the encapsulation, using a custom way to rewrite the underlying structure, you can better understand the net/http package.